How to construct Scalable Applications for a Developer By Gustavo Woltmann
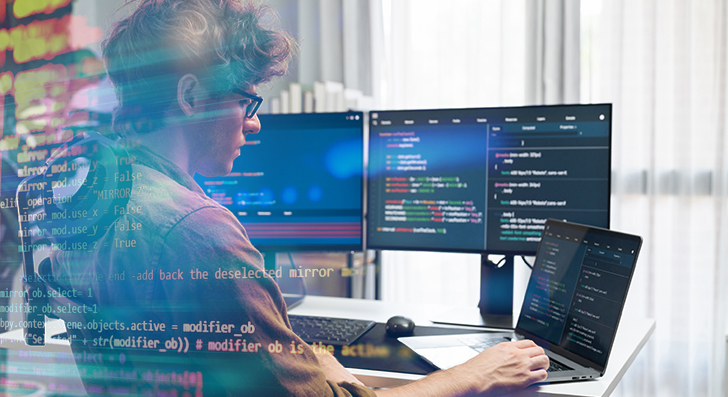
Scalability means your application can take care of growth—extra people, a lot more information, and more targeted visitors—devoid of breaking. Like a developer, building with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook to help you start out by Gustavo Woltmann.
Design and style for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it should be section of one's approach from the beginning. Lots of apps fail whenever they develop rapid simply because the initial design can’t take care of the additional load. Like a developer, you have to Consider early regarding how your program will behave stressed.
Start by planning your architecture to generally be flexible. Prevent monolithic codebases in which all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns break your application into lesser, independent components. Each module or support can scale By itself without the need of affecting The entire technique.
Also, contemplate your databases from working day 1. Will it have to have to handle a million customers or maybe 100? Pick the suitable type—relational or NoSQL—according to how your info will mature. Plan for sharding, indexing, and backups early, even if you don’t want them nevertheless.
A different vital issue is to avoid hardcoding assumptions. Don’t create code that only functions underneath latest disorders. Give thought to what would happen if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure styles that aid scaling, like information queues or party-pushed devices. These assistance your application cope with additional requests without getting overloaded.
When you Establish with scalability in your mind, you are not just getting ready for success—you might be lessening long run problems. A very well-planned method is easier to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Database
Picking out the proper database is usually a essential Portion of building scalable purposes. Not all databases are created the identical, and utilizing the Mistaken you can slow you down or simply lead to failures as your app grows.
Get started by comprehension your information. Can it be very structured, like rows in a desk? If Indeed, a relational databases like PostgreSQL or MySQL is a great match. These are definitely sturdy with relationships, transactions, and regularity. In addition they assist scaling methods like read replicas, indexing, and partitioning to manage more targeted traffic and information.
If the information is much more flexible—like consumer exercise logs, item catalogs, or paperwork—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more quickly.
Also, think about your read through and generate designs. Are you carrying out numerous reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases which can take care of superior write throughput, and even function-centered data storage methods like Apache Kafka (for short term knowledge streams).
It’s also good to think ahead. You may not will need Highly developed scaling features now, but choosing a database that supports them indicates you gained’t want to change later on.
Use indexing to hurry up queries. Keep away from unnecessary joins. Normalize or denormalize your information according to your accessibility patterns. And often check database efficiency while you expand.
In a nutshell, the correct databases will depend on your application’s construction, pace requirements, and how you anticipate it to grow. Take time to select sensibly—it’ll help save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off adds up. Poorly penned code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by composing clean, very simple code. Prevent repeating logic and remove something pointless. Don’t pick the most advanced Remedy if a simple just one operates. Keep your features brief, concentrated, and simple to test. Use profiling instruments to search out bottlenecks—areas where by your code normally takes as well extensive to run or uses excessive memory.
Subsequent, check out your database queries. These normally slow things down in excess of the code itself. Ensure that Every question only asks for the info you really have to have. Stay away from Find *, which fetches every little thing, and in its place pick specific fields. Use indexes to speed up lookups. And stay clear of performing a lot of joins, especially across substantial tables.
If you recognize a similar information currently being asked for again and again, use caching. Retail store the results briefly working with tools like Redis or Memcached which means you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 records may well crash whenever they have to manage one million.
In short, scalable apps are quickly apps. Maintain your code here restricted, your queries lean, and use caching when necessary. These methods enable your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to take care of extra buyers plus more traffic. If everything goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout a number of servers. As an alternative to a single server carrying out all of the work, the load balancer routes users to distinctive servers based upon availability. This implies no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers request a similar data once more—like an item website page or perhaps a profile—you don’t really need to fetch it from your databases whenever. You are able to provide it in the cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app extra productive.
Use caching for things which don’t alter generally. And always be sure your cache is updated when info does transform.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app handle far more buyers, stay quickly, and Get better from problems. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application develop simply. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to get components or guess future capacity. When visitors raises, you are able to include a lot more sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and safety tools. You can give attention to developing your app instead of running infrastructure.
Containers are A different critical Device. A container deals your app and all the things it ought to run—code, libraries, settings—into one device. This causes it to be simple to maneuver your application involving environments, out of your laptop into the cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your app uses various containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also ensure it is simple to separate portions of your app into expert services. You'll be able to update or scale parts independently, that's great for effectiveness and reliability.
Briefly, making use of cloud and container applications implies you could scale quickly, deploy easily, and Recuperate immediately when difficulties materialize. If you'd like your app to increase without limitations, get started utilizing these instruments early. They save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Watch Everything
In case you don’t observe your application, you won’t know when factors go Erroneous. Monitoring will help the thing is how your application is carrying out, place troubles early, and make improved decisions as your app grows. It’s a crucial Section of setting up scalable systems.
Begin by tracking standard metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you obtain and visualize this data.
Don’t just keep track of your servers—check your app also. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes previously mentioned a limit or perhaps a service goes down, you should get notified immediately. This allows you take care of difficulties rapidly, usually just before customers even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, it is possible to roll it back right before it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without the need of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, monitoring helps you maintain your app reputable and scalable. It’s not just about recognizing failures—it’s about understanding your process and making sure it really works effectively, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By planning carefully, optimizing correctly, and utilizing the correct instruments, you are able to Create applications that develop efficiently without breaking under pressure. Start out small, Feel major, and Develop sensible.